Constant Variables
Test data can be of two types, fixed or variable. If it is fixed, we can easily hard code the test data into our test scripts. But sometimes the fixed test data is also used in so many scripts and if it gets changed then it is a huge task to update all the effected test scripts, for example, the URL of your test application. It remains the same but once you shifted to other environments, you need to change it in all of your test scripts. We can easily place the URL in Text file or Excel file outside our test scripts but Java gives us special feature of creating Constants variables which works exactly the same as Environment and Global variable in QTP.
How to do it...
-
Create a 'New Package' file and name it as “utility“, by right click on the Project and select New > Package.
-
Create a 'New Class' file, by right click on the above-created Package and select New > Class and name it as Constant.
-
Assign keywords in this class to your fixed data for e.g. Url, Username and Password.
package utility;
public class Constant {
public static final String URL = "https://www.store.demoqa.com";
public static final String Username = "testuser_1";
public static final String Password = "Test@123";
}
Constants Variables are declared as public static, so that they can be called in any other methods without instantiate the class.
Constant Variables are declared a final, so that they cannot be changed during the execution.
- SignIn_Class will remain same.
package appModules;
import org.openqa.selenium.WebDriver;
import pageObjects.Home_Page;
import pageObjects.LogIn_Page;
public class SignIn_Action {
public static void Execute(WebDriver driver,String sUsername, String sPassword){
Home_Page.lnk_MyAccount(driver).click();
LogIn_Page.txtbx_UserName(driver).sendKeys(sUsername);
LogIn_Page.txtbx_Password(driver).sendKeys(sPassword);
LogIn_Page.btn_LogIn(driver).click();
}
}
Note: Please visit First Test Case, Page Object Model, Modular Driven & Function Parameters first, in case you are finding it hard to understand the above used SignIn_Action class.
5) Create a New Class and name it as Global_Var_TC by right click on the ‘automationFramework‘ Package and select New > Class. We will be creating all our test cases under this package.
Now pass your Constant Variables (URL, Username, and Password) as arguments to your Execute method of SignIn_Action class in your Global_Var_TC test case.
package automationFramework;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import pageObjects.Home_Page;
import appModules.SignIn_Action;
// Import package utility.*
import utility.Constant;
public class Global_Var_TC {
private static WebDriver driver = null;
public static void main(String[] args) {
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Launch the Online Store Website using Constant Variable
driver.get(Constant.URL);
// Pass Constant Variables as arguments to Execute method
SignIn_Action.Execute(driver,Constant.Username,Constant.Password);
System.out.println("Login Successfully, now it is the time to Log Off buddy.");
Home_Page.lnk_LogOut(driver).click();
driver.quit();
}
}
You will notice that once you type 'Constant' and the moment you press dot, all the variables in the Constant class will display. We can expose variables in order to reduce duplicated code. We are able to call these Constant variables multiple times. This will ensure a better maintainable test code, because we only have to make adjustments and improvements in one particular place.
Your Project explorer window will look like this now.
When the test data is not fixed or if the same test script can be executed with the large amount of test data, we use external files for test data. In next chapter of Data Driven Technique - Apache POI you will see how to set large amount of variable test data for the test scripts.
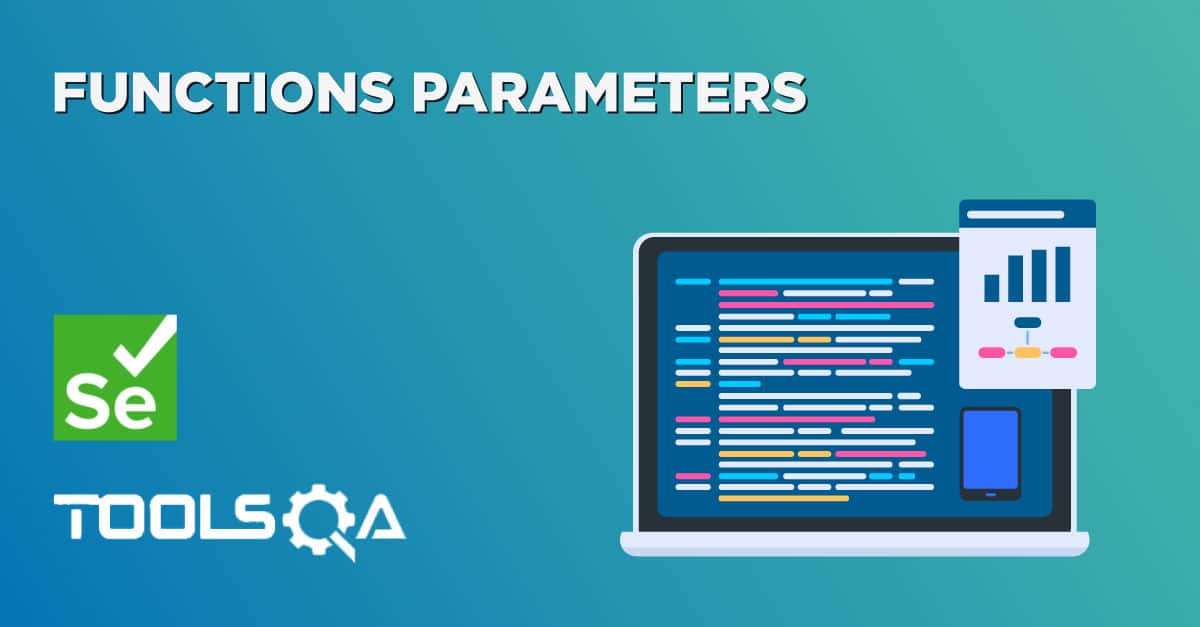
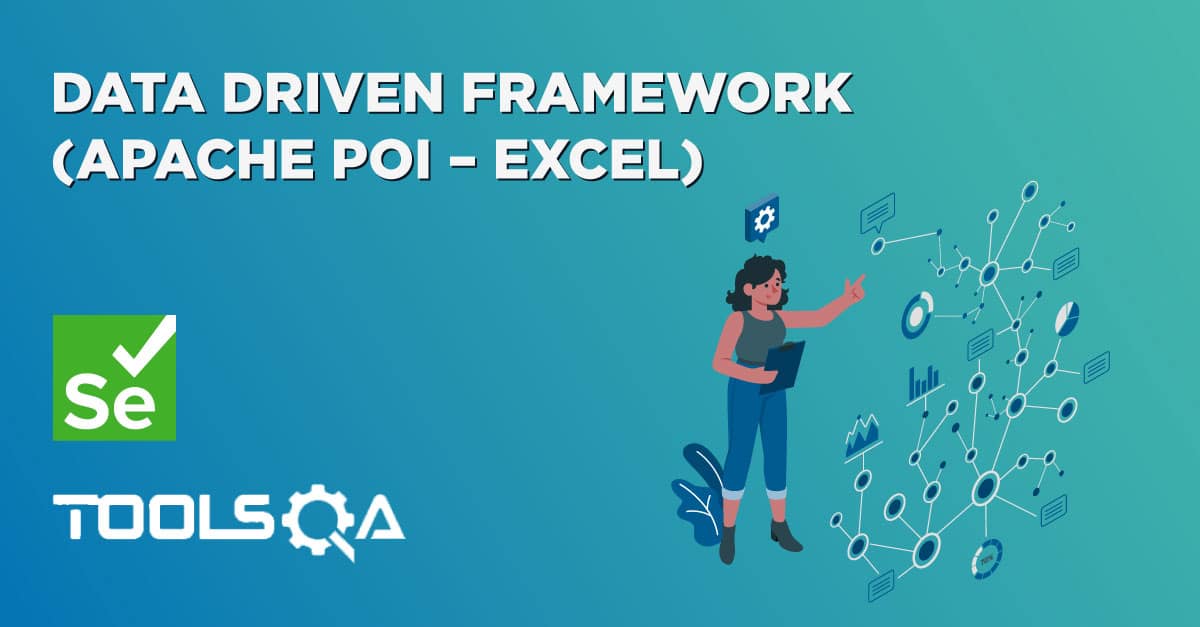