In the previous chapter, we discussed Promises in Protractor. Protractor Browser API is one of the main functionality in Protractor which helps to control the browser behavior or browser operations. In this article, we will discuss topics around Protractor Browser Commands:
- How to open webpage?
- How to get the current webpage title?
- How to get the current webpage URL?
- How to get the current webpage source?
- How to get client session details?
- How to refresh the current page?
- How to restart the browser?
- How to use the protractor for non-angular application?
- How to close the current browser window?
Pre-Requisite:
- Install required packages, If you have not installed please refer to article set up the protractor
- Basic understanding of javascript and typescript
Protractor Browser Commands
Browser API is one of the most used API in Protractor, most of the code always dependent on Browser API. Browser API not just controls the operation of browser methods but also all the webdriver related activity will be taken care of by this.
In Protractor, "browser" is an instance of ProtractorBrowser class. ProtractorBrowser class is a wrapper around WebDriver Interface, which provides additional functionality, in turn, makes protractor easy and fast coding.
The syntax in the tutorial is mentioned in the below format
- Function Name: Name of the function which can be accessed with a browser instance
- Parameter: It's an argument which needs to be passed to get the expected functionality. The parameter data type must be the same as what is expected. For example, in the above screenshot, it is expecting a boolean value true or false (enabled:boolean).
- Return Type: In Protractor all the function returns the type of promise which will be resolved to a specific type. For example Promise
<boolean>
stands for return is a promise which will be resolved to boolean.
Since almost all tests involve protractor browser commands like launch, shutdown, navigation, etc. the test should contain the import browser statement which can be done as below:
import {browser} from 'protractor';
Note: No explicit launch or shutdown of browser is required, In protractor, these actions are handled internally.
Protractor Browser Commands - Navigate to specific URL or webpage in Protractor
Purpose: The get() function in ProtractorBrowser class is used to navigate the specific page.
Syntax: browser.get(destination: string):Promise<any>
Parameter: This command accepts one parameter i.e URL to be navigated. Basically, this URL is a string.
Return: This returns a promise that will be resolved to type any.
Note: Any is data type in typescript. If a variable is declared with <any>
data-type then any type of value can be assigned to that variable.
Code example:
// spec.ts
import { browser} from 'protractor'
describe('Browser API Demo', () => {
it('Navigation Example', () => {
browser.get('https://material.angular.io/');
//OR
browser.get('https://material.angular.io/',10);
});
});
Optionally it accepts the timeout, Once the browser.get() command is executed. It navigates to specified URL and protractor waits for the angular to load, This timeout parameter is the number of milliseconds to wait for Angular to start.
Syntax with Timeout: browser.get(destination: string, timeout?: number)
Protractor Browser Commands - Get current page title in Protractor
Purpose: The getTitle() function in ProtractorBrowser class is used to get the current page title.
Syntax: browser.getTitle() : Promise<string>
Returns: This returns a promise that will be resolved to the current page title as a string
Code Example:
// spec.ts
import { browser} from 'protractor';
describe('Browser API Demo', () => {
it('Get current page title', () => {
browser.get('https://material.angularjs.org')
.then(() => (browser.getTitle()))
.then((title)=>(console.log(title)));
});
});
Protractor Browser Commands - Get current page URL in Protractor
When multiple page navigation is involved in the spec, navigation can be confirmed by fetching current URL using getCurrentUrl() function.
Purpose: The getCurrentUrl() function in ProtractorBrowser class is used to get the current page URL.
Syntax: browser.getCurrentUrl(): Promise<string>
Returns: This returns a promise that will be resolved to the current page title as a string
Code Example:
// spec.ts
import { browser} from 'protractor';
describe('Browser API Demo', () => {
it('Get current page URL', () => {
browser.get('https://material.angularjs.org')
.then(() => (browser.getCurrentUrl()))
.then((url)=>((console.log(url))));
});
});
Protractor Browser Commands - Get the current page source in Protractor
This returns the page source returned is a representation of the underlying DOM. Do not expect it to be formatted or escaped in the same way as the response sent from the webserver.
Purpose: The getPageSource() function in ProtractorBrowser class is used to get the HTML source code of the current page.
Syntax: browser.getPageSource(): Promise<string>
Returns: This returns a promise that will be resolved to the current page title as a string
Code Example:
// spec.ts
import { browser} from 'protractor';
describe('Browser API Demo', () => {
it('Get the current page source', () => {
browser.get('https://material.angularjs.org')
.then((source) => (browser.getPageSource()))
.then((url)=>((console.log(surce))));
});
});
Protractor Browser Commands - Get client session details in Protractor
Purpose: The getSession() function in ProtractorBrowser class is used to get the information of the client session. Information such as sessionId, capability, etc.
Syntax: browser.getSession():Promise<Session>
Returns: This command returns a promise which will be resolved to type Session
Code Example:
// spec.ts
import { browser} from 'protractor';
describe('Browser API Demo', () => {
it('Get client session details', () => {
browser.get('https://material.angularjs.org')
.then(() => (browser.getSession()))
.then((session)=>((console.log(session.getId))));
});
});
Protractor Browser Commands- Refresh the current page in Protractor
This refresh function is used for making full reload of the current page. This method assumes that the current application is angular application and it waits for angular to load before executing the next command. It's not recommended to use if the page is not angular.
Purpose: The refresh() function in ProtractorBrowser class is used to refresh the current page.
Syntax: browser.refresh():Promise<any>
Returns: This command returns the promise which will be resolved to any type.
Code Example:
// spec.ts
import { browser} from 'protractor'
describe('Browser API Demo', () => {
it('Refresh the current page', () => {
browser.get('https://material.angular.io/').then(()=>{
browser.refresh();
});
});
});
Optionally it accepts the timeout, Once the browser.refresh() command is executed. It refreshes the page and protractor waits for the angular to load, This timeout parameter is the number of milliseconds to wait for Angular to start if the angular switch is enabled.
Syntax with Timeout: browser.refresh(timeout?: number)
Protractor Browser Commands - Restart the browser in Protractor
Restart browser is done by closing the current browser instance automatically and creating a new one. But to note, restart function automatically overwrite all the previous settings such as maximize the window, angular switch, etc.
Purpose: The restart() function in ProtractorBrowser class is used to restart the browser instance.
Syntax: browser.restart():Promise<ProtractorBrowser>
Returns: This command returns the promise which will be resolved to ProtractorBrowser type.
Code Example:
// spec.ts
import { browser} from 'protractor'
describe('Browser API Demo', () => {
it('Restart the browser', () => {
browser.get('https://material.angular.io/')
.then(()=>(browser.restart()))
.then(()=>(browser.get('https://material.angular.io/')));
});
});
All cookies and browser settings will be cleared since it restarts with a fresh instance of the browser.
Enable protractor for non-angular application
The default behavior of protractor is that it waits for Angular http and other timeout tasks to complete before interaction. If the webpage is nonangular then the test fails with below message:
“Failed: Angular could not be found on the page https://xyz.com/. If this is not an Angular application, you may need to turn off waiting for Angular”.
In the above case, you must turn off the angular switch. The waitforAngularEnabled() is used to turn off the angular switch in protractor. This function accepts boolean as a parameter. If set to false, Protractor will not wait for Angular http and timeout tasks to complete.
This command can invoke inside the spec or configuration file. Few important points to note here:
- Default is true, protractor always assumes Application Under Test (AUT) is Angular Application.
- When waitForAngularEnabled(false) is used in the configuration file, protractor never waits for the angular page to render. If your application is a combination of Angular and Non-Angular your test may behave flakily.
Purpose: The waitForAngularEnabled() function in ProtractorBrowser class is used to enable or disable angular switch in protractor
Syntax: browser.waitforAngularEnabled(booleanValue:boolean):Promise<boolean>
Returns: This command returns the promise which will be resolved to boolean type.
Code Example: Use waitforAngularEnabled() inside protractor config file
// conf.js
exports.config = {
framework: 'jasmine', //Type of Framework used
seleniumAddress: 'https://localhost:4444/wd/hub',
specs: ['spec.js'], //Name of the Specfile
onPrepare() {
browser.waitForAngularEnabled(false);
}
}
Code Example: Use waitforAngularEnabled() inside spec file
// spec.ts
import { browser} from 'protractor'
describe('Browser API Demo', () => {
browser.waitForAngularEnabled(false); // Doesn't wait for angular application
it('Enable protractor for non-angular application', () => {
browser.get('https://toolsqa.com/');
});
});
Protractor Browser Commands - Close the current browser window in Protractor
Purpose: The close() function in ProtractorBrowser class is used to close the current instance of the browser.
Syntax: browser.close():Promise<void>
Returns: This command returns the promise which will be resolved to void type. (Void means it does not return anything)
Note: Protractor automatically closes after the execution of all tests or specs. However, if needed user can close the current browser window forcibly using close() command.
Code Example:
// spec.ts
import { browser} from 'protractor';
describe('Browser API Demo', () => {
it('Close the current browser window', () => {
browser.get('https://material.angularjs.org') //Navigate to webpage
.then(() => (console.log('Navigated to webpage')))
.then(()=>(browser.close())) //Close the browser
.then(() => (console.log('Browser Closed')))
.then(() => (browser.get('https://material.angularjs.org'))) //Navigate again
});
});
After executing the above line code test fails, as the browser closes and try to navigate to webpage again.
By now you have learned a lot of Protractor Browser Commands, just try to make use of the same. In the next article, we will look at a few more commands of Browser Window.
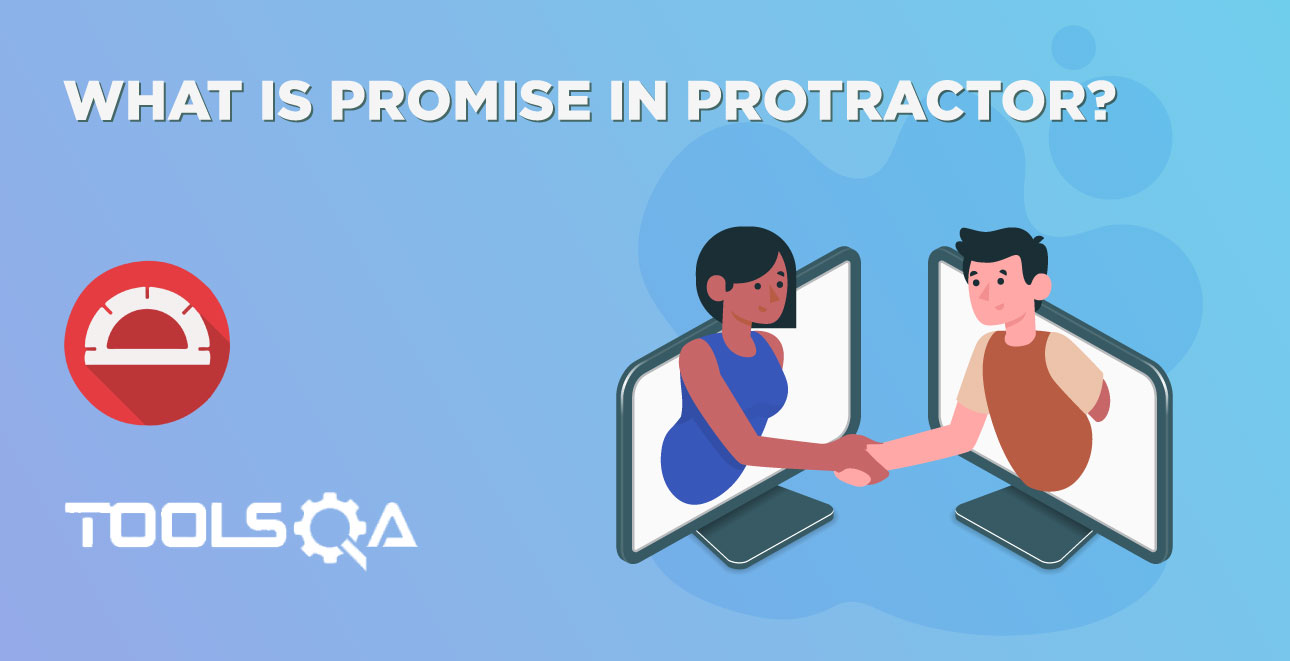
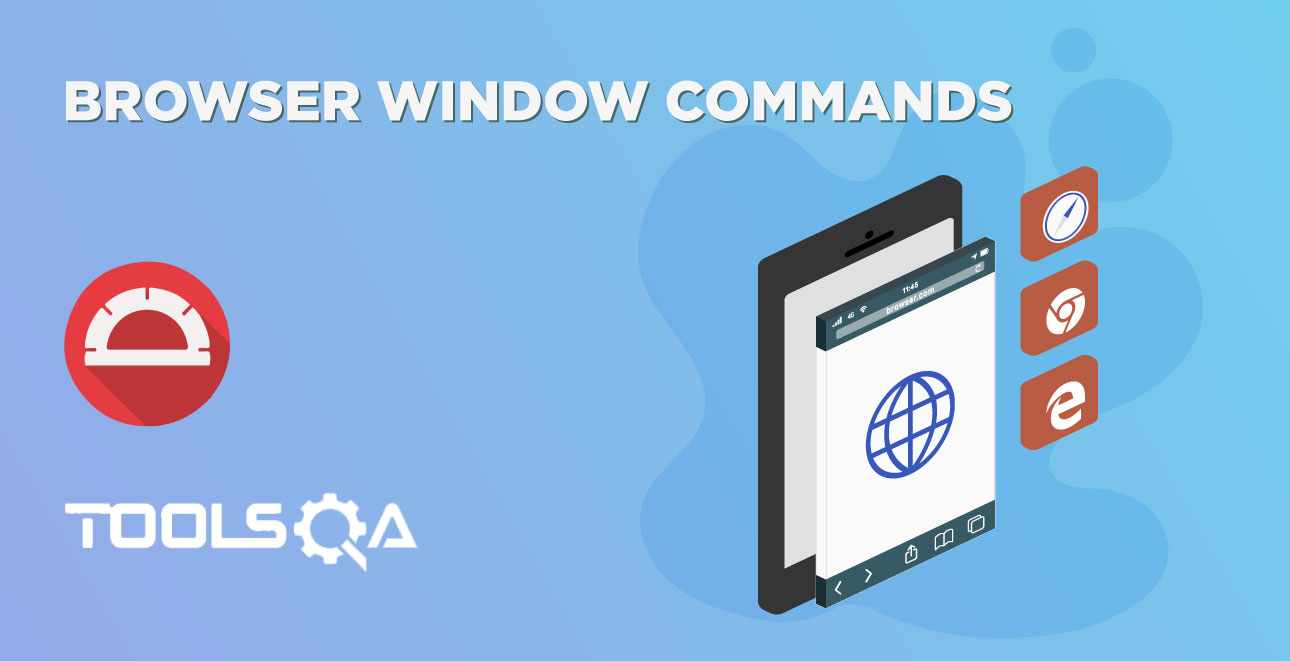